Goal of this project
- Design an algorithm which lets the
XFTimeoutManager
behave in the same timely manner independent of whether it handles one or nn timeouts - Implement the core classes of the XF
- Implement the port classes for following ports: common, idf-qt, idf-stm32
- Test and comment the code of the XF
- The tests provided need to run using Qt and on the Embedded System platform
- Demonstrate the right functioning of the XF by comparing and documenting the output created by the tests running on the Embedded System
Time Algorithm
@startuml
start
:newTime = 0
totalTime = 0
isEnd = it == list.end()
lastTime = 0;
#tomato:if (!isEnd) then (not end)
#tomato:totalTime += it.getRelTicks();
endif
while ( !isEnd && (totalTime <= newTime) ) is (goForward)
:isEnd = (++it == list.end());
:lastTime = totalTime;
#tomato:if (!isEnd) then (not end)
#tomato:totalTime += it.getRelTicks();
endif
endwhile
#tomato:if (!isEnd) then (not end)
#tomato:subRelTicks(newTime- lastTime);
endif
:it.setRelTicks(newTime - lastTime);
:insert(it, newTimeout);
@enduml
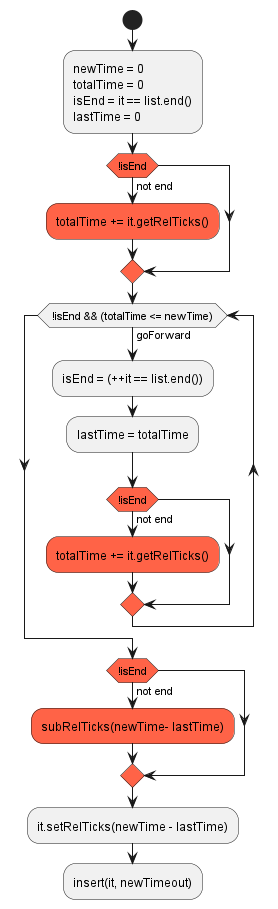
Tests
Test 1
This test launches an instance of the Task01Tm class and produces some output. Optionally, multiple instances of Task01Tm can be created to test the XF.
The Test Factory (TestFactory01) instantiates 2 objects:
- An object of Task01Tm which outputs the text "Say Hello" every second
- An object of Task01Tm which outputs the text "Echo" every half second
Test 2
This test checks again timeout handling and proper termination of a state machine. In case the state machine was statically/globally created, the XF must not delete the object, when requested to terminate the behavior. If the state-machine was created on the heap (dynamic allocation), the XF must delete the state machine upon request to terminate.
Weather or not the state machine should be deleted is handled with the 'deleteOnTerminate()' method provided by the XFReactive interface.
Test 3
This test checks basic event handling in state machines. In this example the StateMachine03 class sends itself an evRestart event to change from one state to another.
Test 4
Tests if timeouts are correctly cancelled. When leaving a state with a transition having a timeout, without the timeout raises, the timeout must be cancelled (unscheduled).
Test 5
With this test multiple timeouts are added to the XFTimeoutManager list at the same time. This tests the way how new timeouts are added in relation to other timeouts (with the same timeout value) already added to the list. For more details how the objects are created, please refere to the implementation of the TestFactory05 class.